Integrating Drag and Drop Functionality in React with Visx
Written on
Introduction to Visx for Drag and Drop
In this article, we will explore how to utilize the Visx library to incorporate stylish curves and segments into our React application.
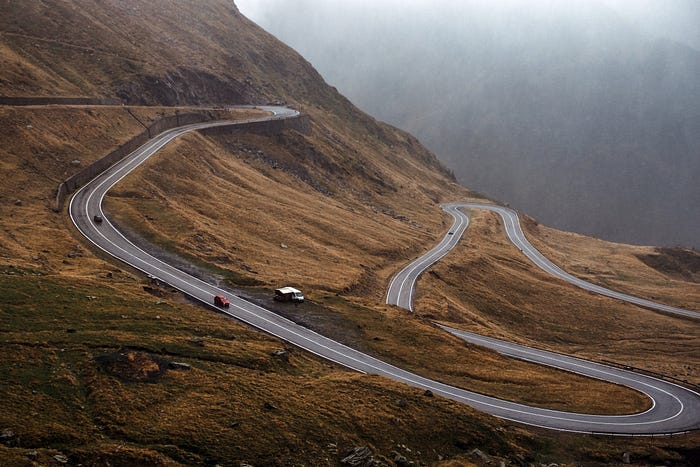
Installing Necessary Packages
To get started with our drag-and-drop feature, we need to install several modules. Execute the following command in your terminal:
npm install @visx/drag @visx/gradient @visx/responsive @visx/scale
Implementing Drag and Drop Functionality
Next, we will write the code to establish the drag-and-drop capability:
import React, { useMemo, useState, useEffect } from "react";
import { scaleOrdinal } from "@visx/scale";
import { LinearGradient } from "@visx/gradient";
import { Drag, raise } from "@visx/drag";
const colors = [
"#025aac",
"#02cff9",
"#02efff",
"#03aeed",
"#0384d7",
"#edfdff",
"#ab31ff",
"#5924d7"
];
const generateCircles = ({ width, height }) =>
new Array(width < 360 ? 40 : 185).fill(1).map((d, i) => {
const radius = 25 - Math.random() * 20;
return {
id: ${i},
radius,
x: Math.round(Math.random() * (width - radius * 2) + radius),
y: Math.round(Math.random() * (height - radius * 2) + radius)
};
});
function Example({ width, height }) {
const [draggingItems, setDraggingItems] = useState([]);
useEffect(() => {
if (width > 10 && height > 10)
setDraggingItems(generateCircles({ width, height }));}, [width, height]);
const colorScale = useMemo(
() =>
scaleOrdinal({
range: colors,
domain: draggingItems.map((d) => d.id)
}),
[width, height]
);
if (draggingItems.length === 0 || width < 10) return null;
return (
<svg width={width} height={height}>
{draggingItems.map((d, i) => (
<Drag key={d.id}
onDragStart={() => setDraggingItems(raise(draggingItems, i))}>{({ dragStart, dragEnd, dragMove, isDragging, x, y, dx, dy }) => (
<circle cx={x} cy={y} r={d.radius} fill={colorScale(d.id)} />)}
</Drag>
))}
</svg>
);
}
export default function App() {
return (
<div>
<Example width={500} height={500} /></div>
);
}
In the above code, the generateCircles function returns an array of circle objects, each having a unique ID, radius, and x/y coordinates for positioning. The Example component manages the draggable items via the draggingItems state, which updates based on the component's dimensions.
The colorScale helps in assigning colors from the predefined color array to each circle. The Drag component serves as a wrapper for the draggable items, and we utilize the provided event handlers to facilitate dragging.
Conclusion
By leveraging the Visx library, we can seamlessly integrate drag-and-drop features into our React application. If you found this article helpful, consider subscribing to our YouTube channel for more related content!
Chapter 2: Additional Video Resources
For further learning, check out the following videos:
This video discusses the creation of a drag-and-drop library using React, focusing on the development process and key features.
In this tutorial, learn to build a complete drag-and-drop interface in just one hour using React Beautiful DND.