How to Upload a Media File Using an API Request
Written on
Chapter 1 Understanding API File Uploads
APIs that operate over HTTP play a crucial role in web applications. With a significant 93.4% adoption rate, REST and similar APIs are likely to remain prevalent for the foreseeable future. Although most API interactions involve data formatted in JSON, Text, or XML, there are scenarios where APIs return PDF or HTML files.
During the development of a tweet scheduling tool, I faced a situation where I needed to include a media file within the body of a POST API request. The most commonly recommended approach I found was to transmit the file by itself within the request body, utilizing it as a MultipartFile. According to Spring, MultipartFile is defined as:
A representation of an uploaded file received in a multipart request.
However, my situation required sending additional parameters. Specifically, I needed to transmit three parameters as JSON and one as a file. The most effective method for handling request bodies containing multiple data types is to treat them as Multipart requests.
In an API, you can consume a multipart request media type as follows:
consumes = {"multipart/form-data"}
According to Swagger, a Multipart request is described as:
Multipart requests combine one or more sets of data into a single body, separated by boundaries. They are typically utilized for file uploads and for sending various types of data in one request (for instance, a file alongside a JSON object).
For transactions that involve substantial amounts of data, we use multipart/form-data. Conversely, for more common applications, we utilize the media type application/x-www-form-urlencoded (Definition from StackOverflow).
In the Spring framework, we typically read the request body with the @RequestBody annotation; however, this reads the entire request body as a single entity. For key-value formatted data, we need to utilize a different annotation: @ModelAttribute.
@PostMapping(path = "/save/media", consumes = {"multipart/form-data" }, produces = "application/json")
public ResponseEntity<Void> samplePost(@ModelAttribute SampleRequest req) {}
We can define the request object as a POJO:
public class CreateTweetRequest {
private String content;
private String name;
private MultipartFile img;
private boolean hasAttachment;
}
To test this API using Postman, select the form-data option under the Body tab.
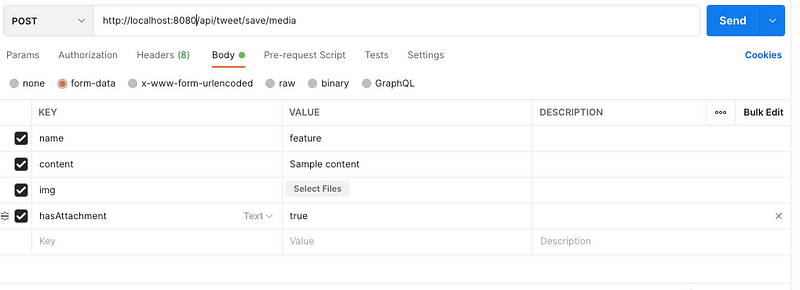
When using form-data for key-value pairs, you can choose between Text or File from the dropdown in the key fields. For the key labeled "Img," select "File" and then click "Select Files" to upload your media file.

Next, we will read the MultipartFile and save it to a designated location within our project:
String orgName = request.getImg().getOriginalFilename();
String fileExt = orgName.split("\.")[1];
Path filepath = Paths.get("src/main/resources/images/", request.getName() + "." + fileExt);
request.getImg().transferTo(filepath);
You can retrieve the original name of the file sent to the POST API using getOriginalFilename(). To transfer the file to the specified path, utilize the transferTo(<filepath>) method.
To establish the maximum allowable file size for uploads to our API, it is necessary to configure the properties in the YAML or application.properties file. If these properties are not set, the POST API call will fail, as the server will be unable to process the size of the transmitted data.
Multipart Configuration
spring.servlet.multipart.enabled=true
spring.servlet.multipart.file-size-threshold=2KB
spring.servlet.multipart.max-file-size=200MB
spring.servlet.multipart.max-request-size=215MB
Conclusion
In conclusion, we have learned how to transfer a file from the client to the Server API within a Spring-based application. The complete code is available on GitHub. If you appreciate my work, please consider giving the repository a star.
Chapter 2 Practical Demonstrations
In this video titled "Upload a File via POST Request | Postman Level Up," you will learn how to upload files using Postman effectively.
The second video, "POST form-data file upload + JSON," demonstrates the process of uploading files alongside JSON data in a POST request.