Enhancing Our E-Commerce App: Integrating Checkout with Vue.js
Written on
Introduction
In this tutorial, we'll explore how to implement a payment gateway using Stripe in our demo e-commerce application built with Vue.js for the frontend and Java with Spring Boot for the backend. Be sure to check the first part of this front-end series for a solid foundation.
Creating the Frontend for Our E-Commerce Application with Vue.js
We're developing a full-fledged e-commerce application from the ground up. In this session, we will add the functionality for products.
A payment gateway is vital for any e-commerce application. In our previous tutorial, we focused on integrating the payment gateway using Spring Boot along with Stripe. Now, we will work on the frontend aspect, which involves handling cart items and card information.
If you’d like to review the backend implementation for the payment gateway, you can find the details in the following link.
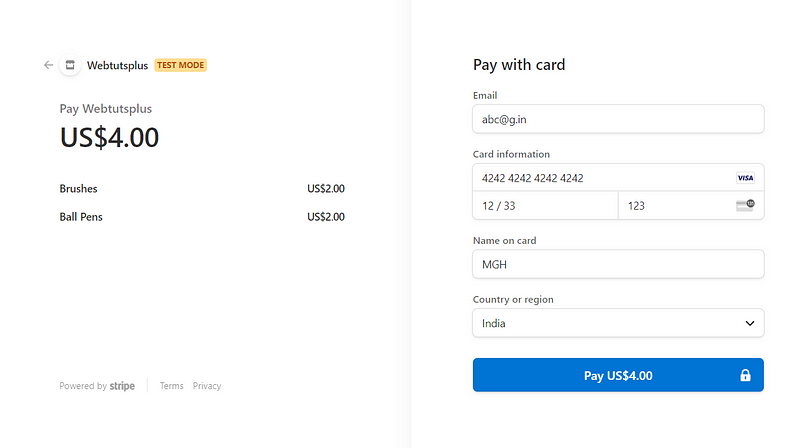
Live Demo
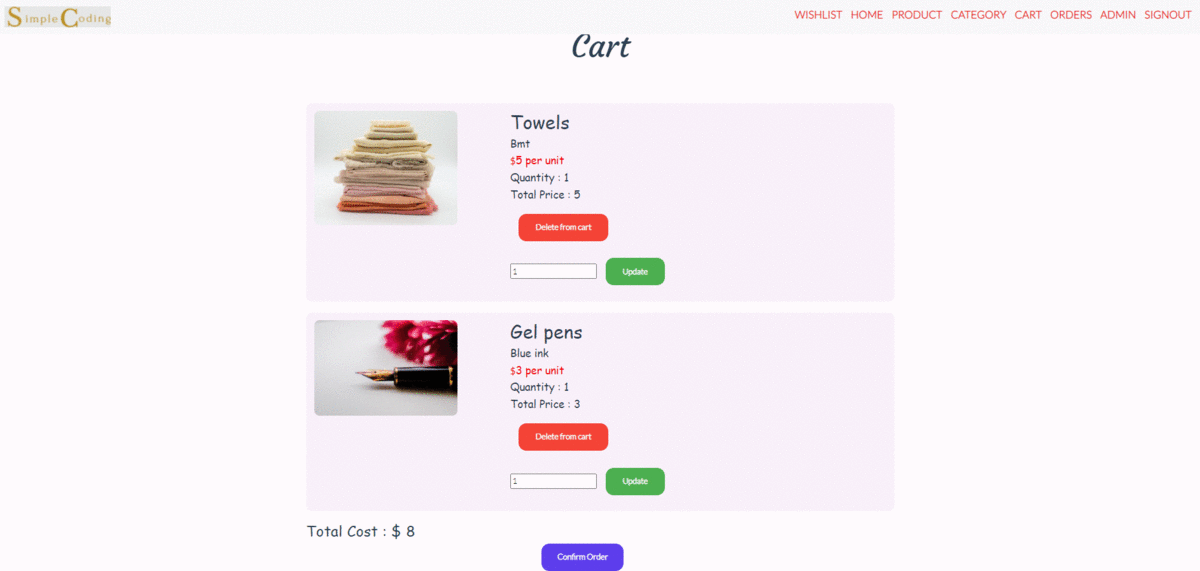
Prerequisites
- Familiarity with Vue.js
- Visual Studio Code (Recommended)
- A reliable web browser (Chrome is preferred)
API Utilized
Here are the key methods we'll be using:
- getCartItems: This GET method retrieves cart items and stores them in an array, which will be sent to the backend API using Axios.
- checkoutList: This POST method sends the cart items array as the request body, generating the session ID.
- placeOrder: This POST method records the order in the database upon successful payment, using the logged-in user's token and the generated session ID.
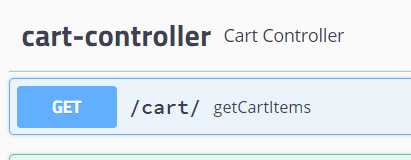
Integrating Stripe Payment Processing
Stripe is renowned for its online payment processing capabilities. Recently, they launched Stripe Elements, a library of pre-built UI components that simplify the creation of customized checkout flows. We will utilize Stripe.js to establish our payment gateway.
To get started, you'll need a Stripe test API key. Create an account on Stripe and retrieve your API key from the dashboard. Store the key in an environment variable by creating a .env file in the source directory.
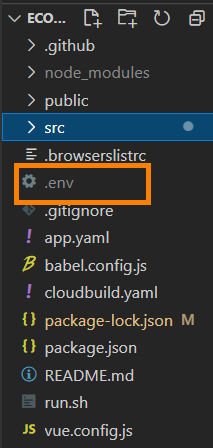
Here's the workflow for this tutorial:
- Declare necessary data variables.
- Install Stripe.
- Initialize and configure Stripe Elements.
- Fetch cart items.
- Send cart items to the backend API and receive the session ID.
- Redirect users based on payment status.
Now, let's dive into coding.
Creating the Checkout Component
Create a folder named Checkout, and within it, create a Vue file called Checkout.vue to manage the payment gateway logic.
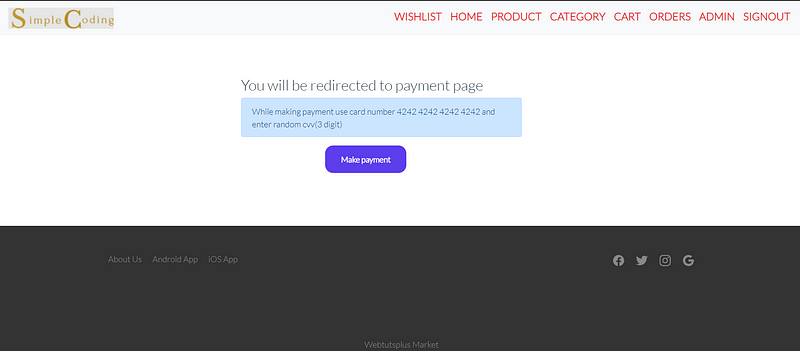
Variable Declaration
- stripeAPIToken: stores the API key.
- stripe: represents a Stripe element.
- token: holds the user's token.
- sessionId: contains the generated session ID.
- checkoutBodyArray: the response body sent to the backend API.
Installing Stripe
To include Stripe.js, implement the includeStripe method, which dynamically loads the Stripe library.
Fetching Cart Items
Implement the getAllItems method, which retrieves cart items via the /cart API and stores them in checkoutBodyArray.
Sending Cart Items to the Backend
The function goToCheckout will call the Axios POST method to send the cart items array to the backend API, which will return a session ID.
Redirecting Users
After receiving the session ID, use Stripe's redirectToCheckout method to navigate the user to a secure payment page.
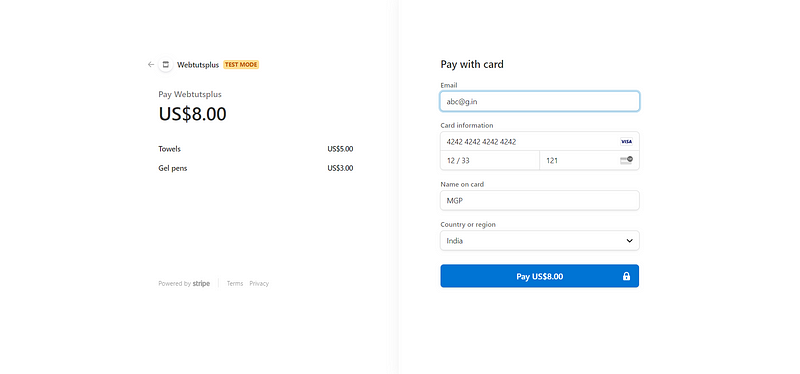
Handling Payment Success or Failure
Once the payment process concludes, we need to manage the outcome. If the payment succeeds, we will log the order in the database; if it fails, we will not record the order and direct the user to the order history page.
In the previous backend tutorial, we set the success and failure URLs.
To handle these outcomes, create a helper folder, and within it, a payment folder containing two Vue components: Failed.vue and Success.vue.
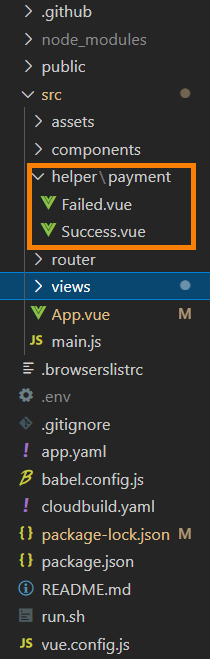
Success Component
- Define data variables for token and session-id, and implement a method to save the current order to the database.
Failure Component
- Remove the session ID from local storage, avoiding the order's recording, and navigate the user to the order history page, showing past orders but not the current one.
Congratulations!
You've successfully integrated the frontend for a payment gateway using Vue.js and Stripe! You can find the complete code on GitHub.
Video Tutorials
Below are some useful video tutorials to enhance your understanding of building an e-commerce application with Vue.js:
Vue.js E-Commerce App Tutorial: Shopping cart frontend
This video covers the foundational aspects of creating a shopping cart frontend with Vue.js.
Vue.js E-Commerce App Tutorial: Shopping cart frontend (part 2)
A continuation that dives deeper into the shopping cart functionality.