Simplified DLL Execution: An Innovative Approach
Written on
Chapter 1: Introduction to DLL Execution
In this article, we’re thrilled to introduce a new, simplified strategy for working with Dynamic Link Libraries (DLLs). Have you ever struggled with the intricacies of injecting DLLs into other processes? If that sounds familiar, you're in luck! We're unveiling a transformative approach that will make DLL execution straightforward and efficient.
Traditionally, developers often faced the daunting task of injecting DLLs into running processes to enable specific features. While this method can be effective, it also brings challenges such as compatibility issues and security risks. Today, we will explore a more efficient method that eliminates these hurdles.
This article focuses on loading DLLs directly into the initial binary using the LoadLibrary function in C++. This clever technique allows you to execute a DLL seamlessly within your application, eliminating the need for cumbersome injections into external processes. By integrating the LoadLibrary function into your C++ code, you can fully leverage the advantages of DLLs while simplifying the process.
The Benefits of Using LoadLibrary
- Simplified Implementation: Unlike traditional DLL injection techniques that often require complex steps and coding, the LoadLibrary function allows for a more straightforward implementation. With only a few lines of C++ code, you can easily load and execute a DLL, significantly reducing development time.
- Enhanced Compatibility: DLL injection can lead to compatibility issues, especially across different operating systems and architectures. LoadLibrary, however, provides a more stable and portable solution, ensuring smooth execution across multiple platforms.
- Reduced Security Risks: Modifying the memory of other processes via DLL injection poses security risks. By using LoadLibrary within your application, you mitigate these concerns, fostering a safer environment for your software.
- Easier Debugging and Maintenance: Integrating DLL execution directly into your C++ code simplifies debugging and maintenance. You can manage DLL dependencies, track issues, and implement updates more effectively without navigating the complexities of external injections.
Code Example
#include <windows.h>
int main() {
HMODULE evilDll = LoadLibraryA("evil.dll");
if (evilDll) {
printf("evil.dll loaded!n");
FreeLibrary(evilDll);
} else {
printf("Failed to load library :(n");}
return 0;
}
This C++ snippet illustrates how to use the Windows API functions LoadLibrary and FreeLibrary to dynamically load a DLL named "evil.dll" and subsequently unload it. Let’s break it down step by step:
- #include <windows.h>: This includes the Windows API header, which provides access to numerous functions and data types essential for Windows programming.
- int main() { ... }: This marks the entry point of the program.
- HMODULE evilDll = LoadLibraryA("evil.dll");: This line attempts to load "evil.dll" into the program’s address space. If successful, it returns a handle; otherwise, it returns NULL.
- The conditional check determines whether the DLL was loaded successfully, printing an appropriate message to the console.
- FreeLibrary(evilDll);: This line unloads the DLL, ensuring that resources are freed and preventing memory leaks.
In summary, this code attempts to load "evil.dll" using LoadLibrary. If the loading is successful, it displays a success message, and then it unloads the DLL. If it fails, an error message is shown. Note that the functionality of "evil.dll" is not included here; you can substitute it with any DLL you wish to load.
Chapter 2: Creating the DLL
Below is the code for creating the DLL itself:
#include <windows.h>
#pragma comment(lib, "user32.lib")
BOOL APIENTRY DllMain(HMODULE hModule, DWORD nReason, LPVOID lpReserved) {
switch (nReason) {
case DLL_PROCESS_ATTACH:
MessageBox(NULL, "S12!", "MessageBox by S12", MB_OK);
break;
case DLL_PROCESS_DETACH:
case DLL_THREAD_ATTACH:
case DLL_THREAD_DETACH:
break;}
return TRUE;
}
This code defines a DLL and its entry point via the DllMain function. When attached to a process, it displays a message box. Here’s a breakdown of the code:
- #include <windows.h>: This includes the necessary Windows API header.
- #pragma comment(lib, "user32.lib"): This directive automatically links the user32.lib library, which contains functions for user interface operations, including MessageBox.
- The DllMain function is automatically invoked when the DLL is loaded or unloaded, and it handles various events related to the process.
Proof of Concept
To compile both files, use the following command:
x86_64-w64-mingw32-g++ -O2 executeDLL.cpp -o executeDLL -I/usr/share/mingw-w64/include -L/usr/lib -s -ffunction-sections -fdata-sections -Wno-write-strings -fno-exceptions -fmerge-all-constants -static-libstdc++ -static-libgcc -fpermissive -Wnarrowing -fexceptions

To create the DLL, use:
x86_64-w64-mingw32-gcc -shared -o evil.dll dll.cpp -fpermissive

Now, let’s execute the program:
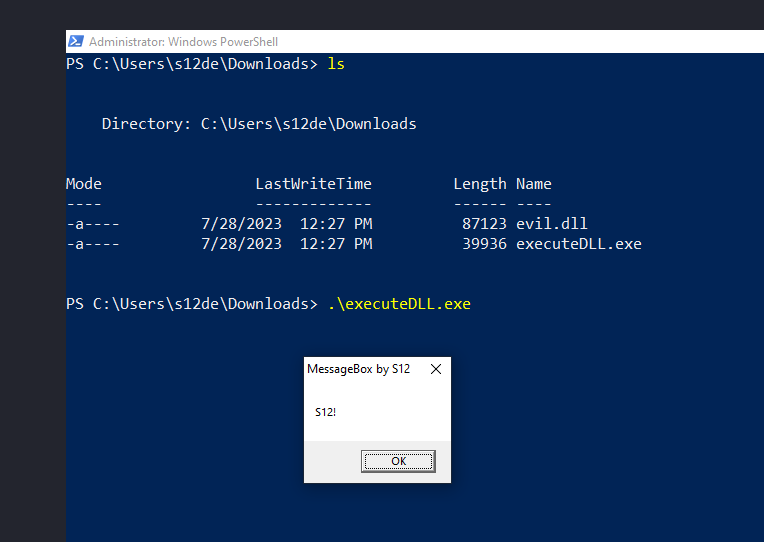

Everything works flawlessly!
Conclusions
In conclusion, this article delved into the fascinating realm of DLL execution, showcasing a revolutionary method to simplify the process. Traditional methods often involved complex DLL injections that could lead to compatibility and security issues. However, our approach utilizing the LoadLibrary function provides a more efficient and secure alternative.
The benefits of LoadLibrary are evident:
- Simplified Implementation: Requires minimal code, saving time and effort.
- Enhanced Compatibility: Ensures robust execution across various platforms.
- Reduced Security Risks: Minimizes security concerns linked to process memory modification.
- Improved Debugging and Maintenance: Facilitates easier management of DLL dependencies and updates.
We hope this article has illuminated a more effective and straightforward method for working with DLLs, empowering developers to enhance their projects while maintaining simplicity and security.
We deeply appreciate our readers for their support. If you found this article valuable and wish to contribute further, consider becoming a member or sharing our project. Your encouragement drives our commitment to delivering high-quality content and insights.
Thank you for joining us on this journey, and we look forward to providing more exciting and informative articles in the future!
If you enjoy my content and would like to help me take this project to the next level, consider becoming a member by donating a monthly subscription. Your support will help me continue to create high-quality content. Thank you for your generosity!
The first video title is [31] Malware Theory - DLL Execution - YouTube. This video provides a comprehensive overview of DLL execution and its implications in malware development.
The second video title is HOW TO EXECUTE MALWARE FROM DLL FILE - YouTube. This tutorial walks viewers through the steps of executing malware from a DLL file, offering insights into practical applications.