Mastering Fastify for Back-End Development: A Comprehensive Guide
Written on
Chapter 1: Introduction to Fastify
Fastify is a lightweight framework for Node.js, designed for building back-end web applications. In this guide, we will explore various features of Fastify that streamline back-end development.
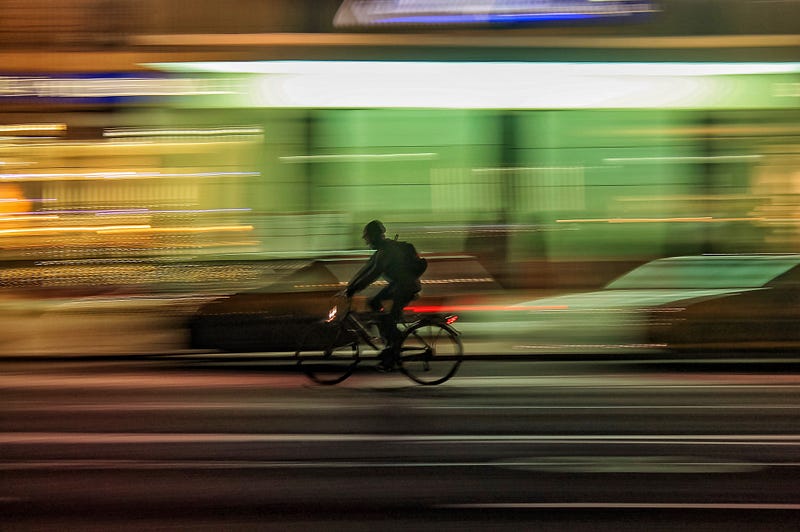
Section 1.1: Closing the Server
To gracefully shut down the Fastify server, you can utilize the fastify.close method. Here’s how you can implement it:
const fastify = require('fastify')()
fastify.get('/', (request, reply) => {
reply.send('hello world')
})
fastify.ready(err => {
if (err) throw err
})
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0')} catch (err) {
fastify.log.error(err)
await fastify.close()
process.exit(1)
}
}
start()
This code demonstrates how to call fastify.close without any arguments, which will return a promise.
Section 1.2: Adding Route Prefixes
You can enhance your routes by adding prefixes using the prefix option. Below is an example:
const fastify = require('fastify')()
fastify.register(function (instance, opts, done) {
instance.get('/foo', function (request, reply) {
request.log.info('prefix: %s', instance.prefix)
reply.send({ prefix: instance.prefix })
})
instance.register(function (instance, opts, done) {
instance.get('/bar', function (request, reply) {
request.log.info('prefix: %s', instance.prefix)
reply.send({ prefix: instance.prefix })
})
done()
}, { prefix: '/v2' })
done()
}, { prefix: '/v1' })
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0')} catch (err) {
fastify.log.error(err)
process.exit(1)
}
}
start()
When accessing /v1/v2/bar, the response will be:
{
"prefix": "/v1/v2"
}
And for /v1/foo, you will see:
{
"prefix": "/v1"
}
Chapter 2: Configuring Reply Serialization
Section 2.1: Setting Reply Serializers
To customize the response format for all routes, you can utilize the setReplySerializer method. Here’s an example:
const fastify = require('fastify')()
fastify.setReplySerializer(function (payload, statusCode) {
return status: ${statusCode}, content: ${payload}
})
fastify.get('/', async (request, reply) => {
return 'hello world'
})
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0')} catch (err) {
fastify.log.error(err)
await fastify.close()
process.exit(1)
}
}
start()
This code snippet illustrates how to integrate a serializer into your Fastify application.
Section 2.2: Handling Not Found Errors
You can define a handler for 404 errors as follows:
const fastify = require('fastify')()
fastify.setNotFoundHandler({
preValidation: (req, reply, done) => {
done()},
preHandler: (req, reply, done) => {
done()}
}, function (request, reply) {
reply.send('not found')
})
fastify.get('/', async (request, reply) => {
return 'hello world'
})
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0')} catch (err) {
fastify.log.error(err)
await fastify.close()
process.exit(1)
}
}
start()
You can also apply a not-found handler to a specific set of routes, as shown here:
const fastify = require('fastify')()
fastify.register(function (instance, options, done) {
instance.setNotFoundHandler(function (request, reply) {
return reply.send('not found')})
done()
}, { prefix: '/v1' })
fastify.get('/', async (request, reply) => {
return 'hello world'
})
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0')} catch (err) {
fastify.log.error(err)
await fastify.close()
process.exit(1)
}
}
start()
Conclusion
With Fastify, you can effectively close the server, implement route prefixes, manage not-found handlers, and establish reply serialization. This framework provides a robust foundation for building efficient back-end applications.