Mastering Event Handling with React and TypeScript
Written on
Chapter 1: Introduction to Event Handling
Welcome, everyone!
Handling events is a fundamental aspect of working with React. However, when you start incorporating TypeScript into your React applications, event management can become a bit challenging, often leading you to use the 'any' type just to keep things moving. In this guide, we will explore how to simplify your event handling experience.
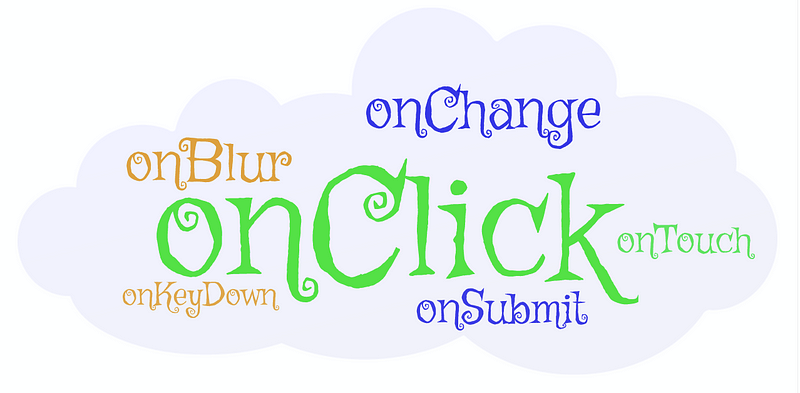
Benefits of Using TypeScript for Event Management
As a statically typed language, TypeScript helps catch errors early in the development process, well before your application goes live. Here are some compelling reasons to adopt TypeScript:
- Enhanced Readability: TypeScript makes your code more comprehensible and easier to maintain.
- IntelliSense Support: Editors like VS Code provide excellent IntelliSense support, aiding developers in their coding tasks.
- Increased Confidence: The use of types boosts your confidence in the code's integrity.
- Growing Library Support: With increasing compatibility of TypeScript across libraries, integrating it with React has become even more beneficial.
In this article, I will demonstrate how to effectively manage JavaScript events within the React and TypeScript framework. I’ll share snippets that will be particularly useful for those new to TypeScript in React. Below are some commonly used event handlers in React along with my insights.
Before we dive deeper, let's understand how to type functions in TypeScript.
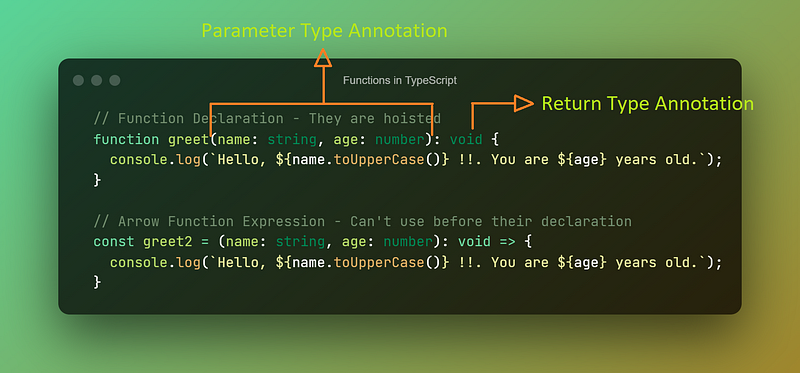
Understanding Function Types
In most cases, you won't need to specify a return type annotation, as TypeScript can infer it from the function's return statements.
Standard Event Type Syntax:
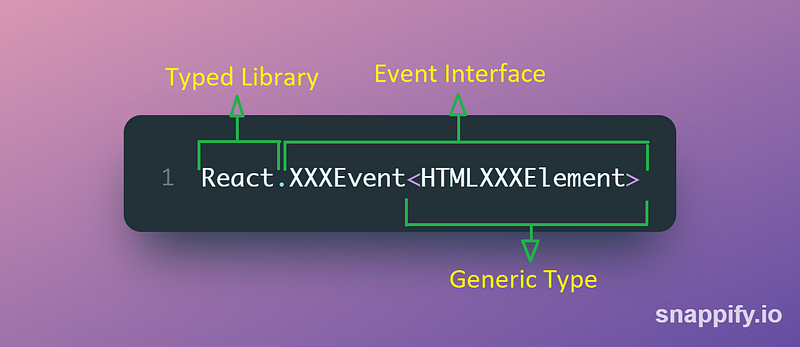
Common Event Types
Click Event:
The Click event is one of the most frequently used events in React, requiring regular handling.
- Trigger: When a user clicks on an HTML element.
- Type: React.MouseEvent<HTMLxxxElement>
- Example:
(Insert example code snippet here)
Change Event:
This event is vital for updating the input state within components, particularly in forms. It provides immediate feedback to enhance user experience.
- Trigger: React Synthetic change event compares the DOM and virtual DOM, firing only when an input change occurs.
- Type: React.ChangeEvent<HTMLxxxElement>
- Example:
(Insert example code snippet here)
Blur Event:
The blur event activates when focus shifts away from an element, useful for validating required conditions and providing feedback.
- Trigger: When focus leaves an element.
- Type: React.FocusEvent<HTMLxxxElement>
- Example:
(Insert example code snippet here)
Submit Event:
This event is triggered upon form submission. By default, forms redirect based on the action attribute. To prevent this, you can use the preventDefault method.
- Trigger: When a form is submitted.
- Type: React.FormEvent<HTMLFormElement>
- Example:
(Insert example code snippet here)
Keydown Event:
The Keydown event handles user inputs via keyboard, enhancing accessibility. The deprecated keypress event can cause confusion, making keydown a preferred choice.
- Trigger: When a key is pressed.
- Type: React.KeyboardEvent<HTMLxxxElement>
- Example:
(Insert example code snippet here)
Fun Fact: If you include <input type="submit" /> or <button type="submit">Submit</button> within a form, you don't need a KeyDown handler to submit the form on pressing the 'Enter' key; this functionality is automatically integrated.
I hope this information simplifies your experience. For further assistance, feel free to explore my React projects on GitHub.
The first video titled "React Crash Course 2024" provides an overview of fundamental React concepts, making it an excellent resource for beginners.
The second video, "React JS Crash Course," dives deeper into React, offering practical examples and best practices for using the library efficiently.
Bonus Tip: If you utilize inline event handlers, TypeScript can infer the event type without requiring additional specifications.
Take care and remember to carve out time for your hobbies!
References:
- Official TypeScript Documentation for React
- React Event Types CheatSheet
- Differences Between Change and Input Events in React
- Form Submission on Enter Key
For more insights, visit PlainEnglish.io. Subscribe to our newsletter for weekly updates. Follow us on Twitter and LinkedIn, and join our Community Discord for more discussions.