A Comprehensive Guide to the Factory Design Pattern in Java
Written on
Chapter 1: Understanding the Factory Method Pattern
The Factory Method pattern is a widely recognized creational design pattern that provides a solution for object creation without needing to specify the precise class of the object being instantiated. This pattern is notably helpful in scenarios where you want to delegate the instantiation process to subclasses or factories.
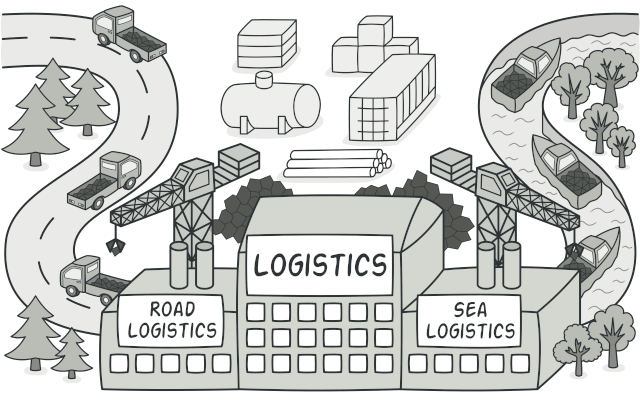
Consider an example involving an OrderService class. This class is responsible for generating an Order object based on specific events. For instance, if a newOrderEvent occurs, it will create an Order using the logic associated with new orders; conversely, if a cancelOrderEvent is triggered, it will generate an Order based on the cancellation logic.
public class OrderService {
private NewOrderCreator newOrderCreator;
private CancelOrderCreator cancelOrderCreator;
private Order order;
// Some Code
public void handleNewOrder(NewOrderEvent event){
order = newOrderCreator.create(event.getDetails());}
public void handleCancelOrder(CancelOrderEvent event){
order = cancelOrderCreator.create(event.getDetails());}
}
However, can we simplify this process further? Rather than predefining the instances, we can implement a factory class that encapsulates the logic for determining which order creator to use.
public class OrderCreatorFactory {
public static OrderCreator getOrderCreator(Event event){
if (event instanceof NewOrderEvent){
return new NewOrderCreator();} else if (event instanceof CancelOrderEvent){
return new CancelOrderCreator();}
return null;
}
}
In this factory class, we abstract the order creators and events into interfaces named OrderCreator and Event. The getOrderCreator method is static, allowing us to invoke it without instantiating the factory itself. Depending on the type of event passed, this method returns the appropriate OrderCreator.
In the revised OrderService class, we no longer have to create instances manually, as this responsibility is delegated to the factory class. Thus, we can refactor our code as follows:
public class OrderService {
private OrderCreatorFactory orderCreatorFactory;
private Order order;
// Some Code
public void handleOrder(Event event){
order = orderCreatorFactory.getOrderCreator(event)
.create(event.getDetails());}
}
Now, we have a unified handleOrder method that can manage both new and canceled orders, thanks to the abstraction provided by the factory. If new order types need to be implemented in the future, we simply update the factory class, enhancing code extensibility and cleanliness.
I trust you found this guide informative. If you share my passion for learning about technology and reflecting on work and life, consider following my channel for ongoing insights and inspirations.
Chapter 2: Video Resources on Factory Design Patterns
Explore the Factory Design Pattern in Java through this insightful video, which walks you through the core concepts and implementation strategies.
In this video, learn about the Abstract Factory Design Pattern in Java, which builds on the principles introduced in the Factory Method pattern.