A Deep Dive into Recommender Systems Using Python
Written on
Introduction to Recommender Systems
Recommender systems, often referred to as recommendation engines, play a crucial role in enhancing our online experiences. They help users discover products to buy, movies to enjoy, music to listen to, and much more. This guide aims to provide a thorough understanding of recommender systems, their various types, and how to implement them with Python.
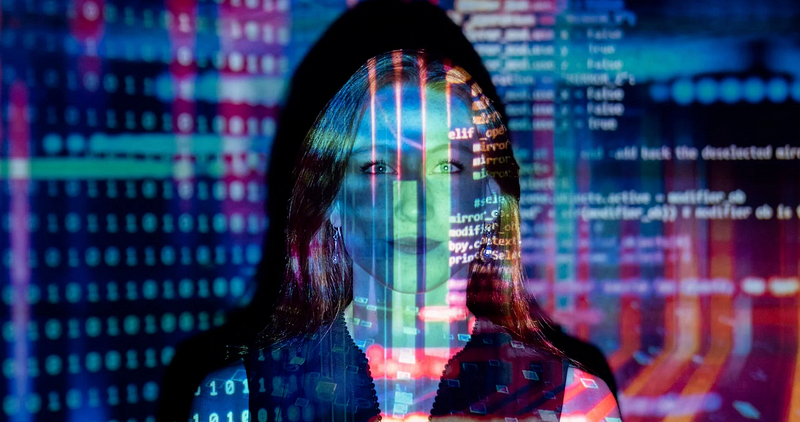
Table of Contents
- Overview of Recommender Systems
- Definition and Significance
- Classification of Recommender Systems
- Content-Based Systems
- Collaborative Filtering Techniques
- Constructing a Content-Based Recommender
- Developing Collaborative Filtering Systems
- Assessing Recommender Systems
- Challenges and Future Directions
Overview of Recommender Systems
Recommender systems belong to a category of information filtering systems designed to predict a user's potential rating or preference for items. They are particularly useful in scenarios where users face a plethora of options, such as selecting books, music, or products.
Significance of Recommender Systems
These systems have a profound impact across various sectors:
- E-commerce: Boost sales by recommending products aligned with users' preferences.
- Streaming Platforms: Keep users engaged by suggesting movies, music, or TV shows.
- Social Media: Enhance user interaction by proposing friends and content to explore.
- News Outlets: Tailor news articles to align with user interests.
Classification of Recommender Systems
Recommender systems can be categorized into several types, including content-based, collaborative filtering, and hybrid systems.
Content-Based Recommender Systems
These systems suggest items that share similarities with what the user has previously shown interest in, focusing on item features. For instance, if a user enjoys action films, the system will propose similar action titles.
Collaborative Filtering
Collaborative filtering methods rely on aggregating and analyzing data about interactions between users and items, further divided into user-based and item-based strategies.
#### User-Based Collaborative Filtering
This technique recommends items by identifying users with comparable preferences and suggesting items they liked, based on the assumption that users with similar tastes in the past will continue to align in the future.
#### Item-Based Collaborative Filtering
Conversely, item-based collaborative filtering recommends items similar to those the user has previously enjoyed, focusing on patterns of interaction between items.
Hybrid Recommender Systems
Hybrid systems combine various recommendation techniques to enhance performance, such as merging content-based and collaborative filtering methods for better accuracy.
Building a Content-Based Recommender System
Understanding Content-Based Filtering
This approach recommends items based on their characteristics and a user's previous behavior. For instance, in a music recommendation scenario, factors like genre, artist, and lyrics may be considered.
Python Code Example: Creating a Movie Recommender
Let’s look at a basic example of a content-based movie recommender system implemented in Python using the Pandas library. This system utilizes movie metadata, such as genres and cast, to provide recommendations based on user input.
import pandas as pd
# Load the dataset
metadata = pd.read_csv('movies_metadata.csv', low_memory=False)
# Create a content-based recommender
def content_based_recommender(title, metadata):
# Select features
features = ['title', 'genres', 'cast', 'director']
# Create a DataFrame with selected features
content = metadata[features]
# Drop rows with missing values
content = content.dropna()
# Lowercase strings and remove spaces
content['title'] = content['title'].str.lower()
content['genres'] = content['genres'].str.lower()
content['cast'] = content['cast'].str.lower()
content['director'] = content['director'].str.lower()
# Combine features into a single string
content['combined'] = content['genres'] + ' ' + content['cast'] + ' ' + content['director']
# Import TfIdfVectorizer from scikit-learn
from sklearn.feature_extraction.text import TfidfVectorizer
# Define a TF-IDF Vectorizer
tfidf = TfidfVectorizer(stop_words='english')
# Construct the TF-IDF matrix
tfidf_matrix = tfidf.fit_transform(content['combined'])
# Import linear_kernel to compute the dot product
from sklearn.metrics.pairwise import linear_kernel
# Compute the cosine similarity matrix
cosine_sim = linear_kernel(tfidf_matrix, tfidf_matrix)
# Get the index of the movie that matches the title
indices = pd.Series(content.index, index=content['title']).drop_duplicates()
idx = indices[title]
# Get the pairwise similarity scores of all movies with that movie
sim_scores = list(enumerate(cosine_sim[idx]))
# Sort movies based on the similarity scores
sim_scores = sorted(sim_scores, key=lambda x: x[1], reverse=True)
# Get the scores of the 10 most similar movies
sim_scores = sim_scores[1:11]
# Get the movie indices
movie_indices = [i[0] for i in sim_scores]
# Return the top 10 most similar movies
return content['title'].iloc[movie_indices]
# Get recommendations for a movie
recommended_movies = content_based_recommender('avatar', metadata)
print(recommended_movies)
This code snippet illustrates a content-based recommender system that takes a movie title as input and suggests films with similar attributes, such as genres, cast, and director.
Building Collaborative Filtering Recommender Systems
User-Based Collaborative Filtering
User-based collaborative filtering predicts user interests by gathering preferences from multiple users, operating on the premise that those who have agreed in the past will likely agree again.
Item-Based Collaborative Filtering
This method focuses on item similarity based on user interactions, somewhat akin to content-based filtering but leveraging user-item interaction trends.
Python Code Example: User-Based Collaborative Filtering
Now, let’s explore a user-based collaborative filtering implementation using the MovieLens dataset.
import pandas as pd
from surprise import Dataset, Reader
from surprise import KNNBasic
from surprise.model_selection import cross_validate
# Load the MovieLens dataset
data = Dataset.load_builtin('ml-100k')
# Create a user-based collaborative filtering model
sim_options = {
'name': 'cosine',
'user_based': True # Compute user similarity
}
# Initialize the KNNBasic algorithm
model = KNNBasic(sim_options=sim_options)
# Perform cross-validation
cross_validate(model, data, measures=['RMSE', 'MAE'], cv=5, verbose=True)
This example utilizes the Surprise library to create a user-based collaborative filtering recommender system and evaluate its effectiveness.
Evaluating Recommender Systems
Evaluation Metrics
Evaluating the performance of recommender systems is essential for gauging their effectiveness. Metrics like Root Mean Squared Error (RMSE) and Mean Absolute Error (MAE) are commonly used to assess the accuracy of recommendations.
Python Code Example: Evaluating a Recommender System
To evaluate the quality of our recommender system, we can leverage Python code to compute evaluation metrics on an actual dataset.
from surprise import accuracy
from surprise.model_selection import train_test_split
# Load the dataset
data = Dataset.load_builtin('ml-100k')
# Split the data into a training set and a test set
trainset, testset = train_test_split(data, test_size=0.25)
# Initialize and fit the model
model = KNNBasic(sim_options=sim_options)
model.fit(trainset)
# Make predictions
predictions = model.test(testset)
# Calculate RMSE
rmse = accuracy.rmse(predictions)
print(f'RMSE: {rmse}')
In this segment, we divide the dataset into training and testing sets, train the recommender model, predict outcomes, and calculate RMSE as a performance measure.
Challenges and Future Directions
Cold Start Problem
The cold start problem arises when a recommender system struggles to make accurate suggestions for new users or items with limited interaction history. Strategies such as content-based recommendations or hybrid models can alleviate this issue.
Scalability
As datasets expand, scalability presents a notable challenge. Utilizing distributed computing frameworks, like Apache Spark, can help manage scalability issues in recommender systems.
Privacy Concerns
Recommender systems often require extensive data collection and analysis. Safeguarding user privacy and adhering to data protection regulations remain significant challenges in this domain.
Deep Learning in Recommender Systems
Emerging techniques like neural collaborative filtering show promise in enhancing the performance of recommender systems by harnessing intricate patterns in user behavior and item characteristics.
Stay tuned for the next section, where we delve into the challenges and future trends in recommender systems.
In this video titled "Building a Recommendation System in Python," you will learn how to create a simple recommendation system using Python. This tutorial covers the foundational concepts and practical implementations.
Another insightful video, "Movie Recommendation System With Python And Pandas: Data Project," walks you through building a movie recommendation system using Python and Pandas, providing a hands-on approach to data science projects.